前言
平时在Vue项目中,最常用的状态管理工具就是Vuex了,而最新的Pinia是尤雨溪强烈推荐的一款Vue状态管理工具,也被认为是下一代Vuex的替代产品。
其优点如下:
- 去除了mutations,只有 state,getters和actions,其中actions支持了同步和异步操作
- 不会像Vuex那样有模块嵌套,Pinia只有store的概念,store之间可以相互使用,互不影响,达到模块扁平化的效果
- 更好地支持ts
- 更好地支持Vue2/Vue3
- 逻辑更加清晰,开发起来更加简单
如何使用
安装
创建并挂载
- 在src目录下新建store目录并在其下面创建index.js文件,代码如下:
1 2 3
| import { createPinia } from 'pinia' const store = createPinia() export default store
|
- 在main.js中引入store并挂载,代码如下:
1 2 3 4 5 6 7
| import { createApp } from 'vue' import App from './App.vue' import store from './store/index'
createApp(App) .use(store) .mount('#app')
|
创建store
在src/store文件夹下创建一个js文件,命名按照需求即可,我这边定义为main.js,代码如下:
1 2 3 4 5 6 7 8 9 10 11
| import { defineStore } from 'pinia' export const mainStore = defineStore('main', { state: () => { return { msg: 'hello', count: 1 } }, actions: {}, getters: {} })
|
其中defineStore的第一个参数为该store的名称,第二个参数为一个对象,包含了该store的state,getters和actions,state改为了函数形式,目的应该是像Vue2 options API中的data类似,避免多个store中定义的属性相互受到影响。
使用store
此处使用Vue3的SFC语法,主要是Pinia更适合Vue3这种组合式API风格,方便演示
回显与修改state
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| <script lang="ts" setup> import { mainStore } from '../store/main' import { storeToRefs } from 'pinia'
const store = mainStore() const { count } = storeToRefs(store) // 单条数据直接修改 const handleAddCount = () => { store.count++ } </script>
<template> <div> <p>{{ store.msg }}</p> <p>{{ count }}</p> <button @click="handleAddCount">+</button> </div> </template>
|
- 使用方法与Vuex基本类似,要使用哪个store,就直接进行引入,非常方便,没那么多层级引用
- 其中,我们使用了Pinia中的storeToRefs方法,此方法能够直接解构出该store的state中的某个值,并且是响应式的;如果我们直接从state上解构,那么解构出的值就不是响应式的了。
- 如果我们要修改state中的值,不能直接去修改解构出的值,得去修改state上对应的属性
使用patch对多条数据直接修改
使用patch的方式对数据进行修改,可以加快修改速度,性能更好。patch 方法可以接受两种类型的参数,对象型和回调函数型。
patch + 对象
patch + 函数 注:使用回调函数型时,回调接收一个state参数,state指代的就是对应store中的state
使用方式如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| <script lang="ts" setup> import { mainStore } from '../store/main' import { storeToRefs } from 'pinia'
const store = mainStore() const { count } = storeToRefs(store) // 使用patch + 对象 const updateWithObj = () => { store.$patch({ msg: store.msg === 'hello' ? 'hello world' : 'hello', count: store.count + 2 }) } // 使用$patch + 回调 const updateWithFun = () => { store.$patch((state) => { state.msg = state.msg === 'hello' ? 'hello world' : 'hello' state.count = state.count + 3 }) } </script>
<template> <div> <p>{{ store.msg }}</p> <p>{{ count }}</p> <button @click="updateWithObj">$patch+对象</button> <button @click="updateWithFun">$patch+回调</button> </div> </template>
|
使用actions
- 在src/store/main.js的actions对象中,添加一个方法,代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| import { defineStore } from 'pinia' export const mainStore = defineStore('main', { state: () => { return { msg: 'hello', count: 1 } }, actions: { changeState() { this.count++ this.msg = this.msg === 'hello' ? 'hello world' : 'hello' } }, getters: {} })
|
- 使用方式为:store.方法名,代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| <script lang="ts" setup> import { mainStore } from '../store/main' import { storeToRefs } from 'pinia'
const store = mainStore() const { count } = storeToRefs(store) // 使用action修改数据 const onActionClick = () => { store.changeState() } </script>
<template> <div> <p>{{ store.msg }}</p> <p>{{ count }}</p> <button @click="onActionClick">使用action</button> </div> </template>
|
使用getters
Pinia中的getter和Vue中的计算属性类似,在获取state之前会进行处理,具有缓存性,如果值没有改变,即使多次调用,实际上也只会调用一次。
- 在src/store/main.js的getters对象中进行添加,代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| import { defineStore } from 'pinia' export const mainStore = defineStore('main', { state: () => { return { msg: 'hello', count: 1 } }, getters: { getState(state) { console.log('getState被调用了'); // getter 中不仅可以传递state直接改变数据,也可以使用this来改变数据 return `${state.msg} + ${this.count}` } } })
|
- 使用方式如下:
1 2 3 4 5 6 7 8 9 10 11 12
| <script lang="ts" setup> import { mainStore } from '../store/main' const store = mainStore() </script>
<template> <div> <p>使用getter获取数据:{{ store.getState }}</p> <p>使用getter获取数据:{{ store.getState }}</p> <p>使用getter获取数据:{{ store.getState }}</p> </div> </template>
|
我们可以看到,即使执行了三遍一样的代码,但最终还是只调用了一次。
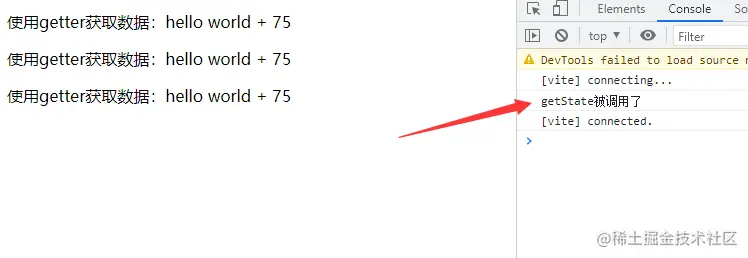
多个store相互调用
在Pinia中,可以在一个store中import引入另外一个store,然后通过调用引入的store方法的形式,获取引入的store的状态。
- 在src/store目录下,新建一个文件.js,代码如下:
1 2 3 4 5 6 7 8 9
| import { defineStore } from 'pinia'
export const userStore = defineStore('user', { state: () => { return { name: '吴同学' } } })
|
- 在需要用到的store中进行引入,并通过getters的方式获取,代码如下:
1 2 3 4 5 6 7 8 9 10
| import { defineStore } from 'pinia' import { userStore } from './user'
export const mainStore = defineStore('main', { getters: { getUserState() { return userStore().name } } })
|
数据持久化
Pinia与Vuex一样,刷新页面后,数据就会重置,有时候我们需要将数据进行持久化存储,我们可以使用pinia-plugin-persist这个插件
安装
1
| npm i pinia-plugin-persist --save
|
使用
- 在src/store/index.js文件夹下,引入并使用,代码如下:
1 2 3 4 5 6 7
| import { createPinia } from 'pinia' import piniaPluginPersist from 'pinia-plugin-persist'
const store = createPinia() store.use(piniaPluginPersist)
export default store
|
- 在对应的store里开启持久化存储
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| import { defineStore } from 'pinia' import { userStore } from './user'
export const mainStore = defineStore('main', { state: () => { return { msg: 'hello', count: 1 } }, // 开启数据缓存 persist: { enabled: true } })
|
更新数据以后,我们就能在浏览器控制台中看到已经将数据存储到了sessionStorage中
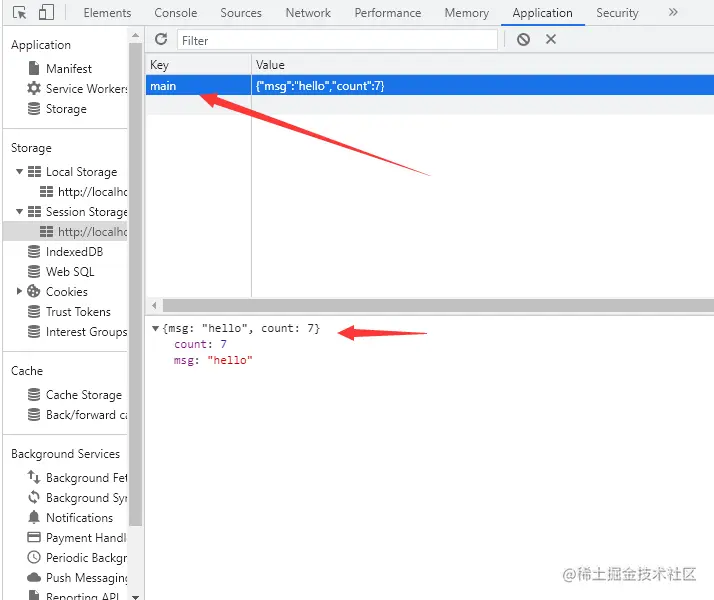
数据默认是存在sessionStorage中的,还会以store的名称作为key。但是我们可以对其修改,并且还可以只持久化部分state中的属性,代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| import { defineStore } from 'pinia' import { userStore } from './user'
export const mainStore = defineStore('main', { state: () => { return { msg: 'hello', count: 1 } }, // 开启数据缓存 persist: { enabled: true, strategies: [ { key: 'mainStore', // 修改存在缓存中的key值 storage: localStorage, // 修改存储方式为localStorage paths:['msg'] // 只持久化msg,此时刷新页面msg数据会被保留,其他state将会重置 } ] } })
|
总结
Pinia就是Vuex的替代产品,相比于Vuex,Pinia更好地兼容了Vue本身,代码更加简洁,开发起来也更加便捷。